CONTROL ARRAYS
Similar to arrays of
variables, you can group a set of controls together as an array. The following
facts apply to control arrays:
- The set of controls that
form a control array must be all of the same type (all textboxes, all
labels, all option buttons, etc.)
- You set up a control array
by naming one or more controls of the same type the same name and
set the Index property of each control in the array to a
non-negative value (i.e., the controls in the control array are usually
indexed from 0 to one less than the number of controls in the array).
- The properties of the
controls in the control array can vary: i.e., some members can be visible
or not, they can be sized differently, they can have different fonts or
colors, etc.
- To refer to a member of a
control array, the syntax is:
ControlName(Index)[.Property]
For
example, to refer to the Text property of the first element of an array of
textboxes called txtField, you would use:
txtField(0).Text
- All the members of the
control array share the same event procedure – for example, if you have a
control array of 10 textboxes call txtField, indexed 0 to 9, you will not
have 10 different GotFocus events – you will just have one that is
shared amongst the 10 members. To differentiate which member of the
control array is being acted upon, VB will automatically pass an Index
parameter to the event procedure. For example, the GotFocus event
procedure for the txtField control array might look like this:
Private
Sub txtField_GotFocus(Index As Integer)
txtField(Index).SelStart = 0
txtField(Index).SelLength = Len(txtField(Index).Text)
End
Sub
-
or -
Private
Sub txtField_GotFocus(Index As Integer)
With txtField(Index)
.SelStart = 0
.SelLength = Len(.Text)
End With
End
Sub
For
events where VB already passes a parameter (for example, the textbox's KeyPress
event where VB passes the KeyAscii parameter), VB will add "Index" as
the first parameter, followed by the parameters that are usually passed to the
event. For example, the procedure header of the KeyPress event of the txtField
control array would look like this:
Private
Sub txtField_KeyPress(Index As Integer, KeyAscii As Integer)
To build a sample
application that uses a control array, perform the following steps:
- Start a new VB project.
Place a command button toward the bottom of the form and set its
properties as follows:
Property Value
(Name) cmdTest
Caption First
At this point
your form should look like this:
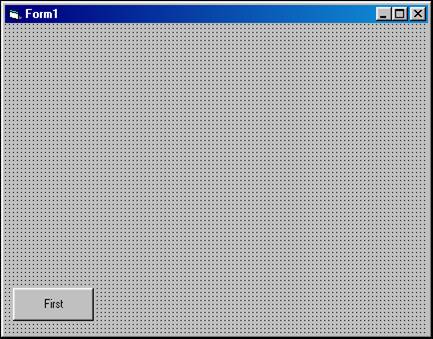
- Click the command button
once to select it. Then Copy it (press Ctrl-C, or Edit ŕ
Copy, or right-click the mouse and choose Copy).
- Click on an open area of
the form and Paste (press Ctrl-V, or Edit ŕ
Paste, or right-click the mouse and choose Paste). The following message
will appear: You already have a control named 'cmdTest'. Do you want
to create a control array? Respond Yes. The pasted control will
appear in the upper left-hand corner of the form. Move the pasted control
toward the bottom, next the original. By answering yes to the prompt, VB
automatically set the Index property of the original command button
to 0 and set the Index of the pasted control to 1.
- Paste two more times (VB will not prompt you any more
now that it knows you want to create a control array), moving the pasted
controls next to the others. Set the Captions of cmdTest(1), (2), and (3)
to "Second", "Third", and "Fourth"
respectively. At this point your form should look like this:
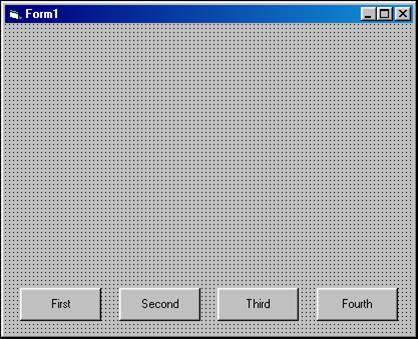
- Place the following code
in the cmdTest_Click event:
Private
Sub cmdTest_Click(Index As Integer)
Print cmdTest(Index).Caption
End
Sub
- Run the project and click
the various buttons in any order. A sample run is shown below:
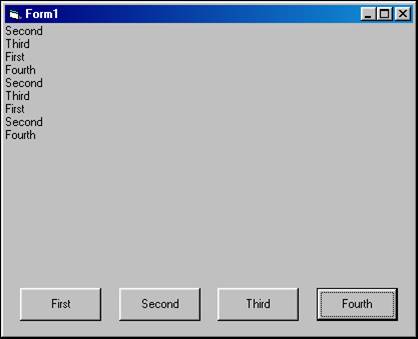
Download the VB project code
for the example above here.